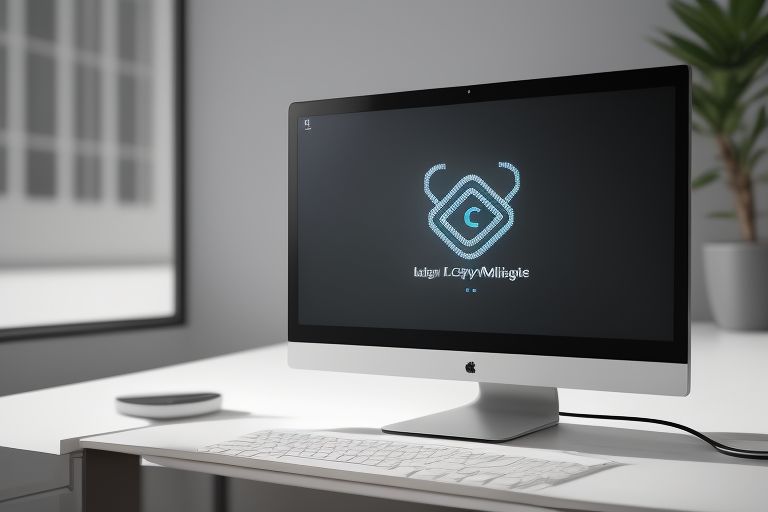
Python has made automating mouse clicks to perform repetitive tasks easy and boosted productivity. PyAutoGUI is one of the most popular tools and is considered a powerful automation tool to simulate mouse and keyboard actions by users. Install it through pip before checking the PyAutoGUI documentation on how to get started. With a simple Python script, one can create functions that relocate the mouse cursor to a certain mouse position, left-clicking by means of the left mouse button, or even dragging things across the screen.
It is that simple to manipulate and control your mouse by a simple debug function that operates on its own in PyAutoGUI: it considers the resolution of the center or current position coordinates of the mouse. You can script pyautogui to scroll up and down on a site, as well as click and type. Supplement these automation scripts with the capabilities of pynput, where your keyboard and mouse clicks would have to listen attentively to every event.
To dive deeper into mouse moves, you can refer to resources on GitHub, where numerous examples and advanced scripts are available. By learning how to use tuples to define pixel positions and understanding every PyAutoGUI command, you can build robust automation solutions. Remember that practice is key, so experiment with different commands and discover how PyAutoGUI works to make your automation projects more effective.
What Is Mouse Click Automation?
Mouse click automation is exactly what it sounds like—using a script or tool to make your mouse click automatically without you physically pressing it. Think of it as a robot finger doing the job for you. It can mimic left or right clicks, double clicks, and even clicks in specific spots on your screen.
Why Automate Mouse Clicks Using Python?
Why Python, you ask? Well, Python is like that super-friendly neighbor who’s always ready to help. It’s easy to understand, widely used, and has loads of free tools you can use.
Whether you’re trying to auto-click in a game or test a piece of software repeatedly, Python gets the job done quickly and cleanly.
How It Works: A Simple Metaphor
Imagine you’re writing a set of instructions for an invisible hand to move your mouse and click things. That’s exactly what we do with code. Like teaching a dog new tricks—but this time, it’s your computer learning the trick of clicking automatically!
Getting Started: What You’ll Need
Before we jump into coding, let’s gather our tools:
- A computer (Windows, Mac, or Linux)
- An internet connection
- Python installed
- A code editor (like VS Code, Notepad++, or even plain Notepad)
- Some curiosity and patience!
Installing Python and pip
First things first, install Python if you haven’t already:
Installing Python is the first step if you haven’t done so yet. Visit https://python.org to download the latest version.
During the installation, ensure you check the box that says Add Python to PATH to simplify command-line access.
- Go to https://python.org
- Download the latest version
- During installation, check the box that says “Add Python to PATH.”
Introducing the PyAutoGUI Library
PyAutoGUI is a powerful library that simplifies controlling the mouse and keyboard. It enables users to automate tasks through mouse movement, simulating user interactions with ease.
By utilizing the library, developers can effectively manage their screen’s actions based on the screen’s resolution and the current mouse position, enhancing productivity with its versatile tools for automating tasks.
Writing Your First Auto Click Script
Creating your first auto click script can be exciting and rewarding. With pyautogui’s simple functions, you can automate mouse clicks effortlessly. Start by installing the library, then import it into your script. Write commands to control the mouse, ensuring to test and tweak settings for optimal performance.
Making It Click Multiple Times
To achieve success, making it click multiple times is essential. This involves understanding your audience’s needs and preferences. Engaging content ensures that users return, fostering loyalty. By consistently delivering value, you encourage repeat interactions, ultimately driving growth and enhancing your brand’s reputation in a competitive landscape.
Adding Time Delays and Loops
To create a drawing program using Python modules, you can utilize the PyAutoGUI function to instantly move the mouse pointer to specific xy coordinates. By importing the module and using PyAutoGUI, you can implement a fail-safe feature that detects the mouse’s current position.
To help simulate drawing a square spiral, employ loops with an integer to control the number of iterations. Incorporating time import allows you to add time delays between actions like left click and button down and then releasing, enhancing the program’s realism.
Use Visual Studio Code as your IDE for writing and executing your programming language scripts. You can also take screenshots of the screen and mouse interactions, and by using the pip, easily download and install necessary image file libraries for enhanced graphics.
Incorporating keyboard keys into your script can provide additional functionality, making the drawing process interactive. By leveraging a dictionary to map commands, you can create a more sophisticated user experience.
Customizing Click Location
Customizing click location involves analyzing user interactions to enhance engagement. By tracking the duration of time users spend on various elements, you can identify which areas attract the most interest. This data enables targeted adjustments, improving user experience and ultimately driving conversions.
Creating a GUI for Your Clicker
Creating a GUI for your Clicker can significantly enhance user experience. Design intuitive buttons and counters to track clicks effortlessly. Incorporate feedback mechanisms, such as sound or visual cues, to engage users further. Ensure that the layout is clean and responsive for optimal interaction across devices.
Safety Tips When Automating Clicks
Automation is powerful, but with great power comes great responsibility.
- Don’t use auto clickers in online games that ban them.
- Always test your scripts in a safe area of your screen.
- Add exit hotkeys using
keyboard
orpyautogui.FAILSAFE = True
for emergencies.
Real-World Use Cases for Auto Clickers
Here are some situations where automating mouse clicks using Python really shines:
- Repeating in-game clicks or farming resources
- Software testing and UI automation
- Clicking “Next” in long online courses
- Speeding up repetitive work tasks
- Auto-accepting pop-ups or prompts
When and Where Not to Use Them
There are also limits to automation. Avoid using auto-clickers.
- In apps where it’s considered cheating (e.g., multiplayer games)
- On websites where clicking repeatedly violates terms
- In ways that could harm your system (e.g., endless loops without stop)
FAQs
1. Can I automate right-clicks and double-clicks using Python?
Yes! With pyautogui.click(button='right')
and pyautogui.doubleClick()
, you can easily simulate those actions.
2. Will auto-click scripts run on all operating systems?
Most of them work on Windows, macOS, and Linux as long as you have Python and PyAutoGUI installed.
3. Is using auto-clickers illegal?Learn how to automate mouse clicks using Python with this easy guide. Perfect for beginners who want to save time and boost productivity.
It depends. They’re fine for personal use, but using them in online games or against terms of service can get you banned.
4. How do I stop an auto-click loop once it starts?
You can stop it with Ctrl+C
in the terminal or use pyautogui.FAILSAFE = True
to move your mouse to the top-left corner and abort.
5. Can I automate clicking on specific images or buttons?
Absolutely! PyAutoGUI can locate images on your screen by clicking them. Try pyautogui.locateOnScreen()
and pyautogui.click()
together.
Final Thoughts and Next Steps
So there you have it! Automating mouse clicks with Python is not just simple—it’s fun and surprisingly useful. You’ve learned how to create your own clicker, control timing, add coordinates, and even build a basic app.
Next, you might explore:
- Automating keyboard presses
- Making advanced GUIs
- Combining mouse and keyboard for full automation
The more you play with it, the more you’ll see how much time and effort you can save. Happy clicking!